Union Quick Start
Union is a development platform for creating connected applications.
In every Union application, all users connect to Union Server using a client application that you, the developer, create. The client application might be a chat, a game, a shared document, or even just a simple multiuser interface-widget, such as the counter below that displays the number of users currently viewing this web page.
Union Server runs in a central location such as Union Cloud or an Internet or LAN host that you provide. Once connected, users are in constant real-time contact, and stay connected for the entire application session. Users communicate with each other by sending messages to, and receiving messages from, Union Server.
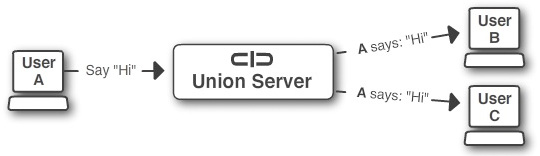
Client applications are typically written using one of Union Platform's official client frameworks, such as Orbiter (a JavaScript framework for creating native Web browser apps) or Reactor (an ActionScript framework for creating Adobe Flash apps). But Union clients can also be custom created in any programming language that has TCP/IP socket or HTTP request capabilities, such as Java, Objective C, Python, C#, and C++. Whatever the language or framework, all Union clients communicate seamlessly with each other. For example, a JavaScript chat client running on an iPhone can send messages to a desktop Java client, or to a Flash client running in Internet Explorer.
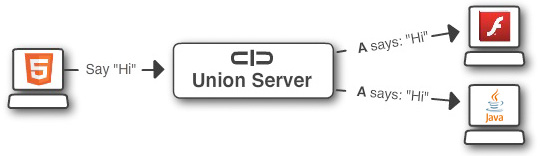
What's The Code Like?
To get a feel for Union application-flow, let's consider a simple Flash chat application named UnionChat. Here's the sequence of events that occurs when two users begin chatting:
- UnionChat starts on computer 'A', and connects to Union Server. Let's call this chat client "Client A". Here's the connection code:
reactor = new Reactor(); reactor.connect("tryunion.com", 80);
- UnionChat starts on computer 'B', and connects to Union Server. Let's call this chat client "Client B".
- Client A asks Union Server to create a "room" for the chat. A "room" is a place for clients to engage in group communication. In this example, we'll name the chat room "Room1". To create "Room1", Client A executes the following code, which sends a "create room request" message to Union Server:
room1 = reactor.getRoomManager().createRoom("Room1");
- Next, client A sends a message to Union Server asking to join "Room1". Here's the code:
room1.join();
- Using the same code, Client B also asks to join Room1. Now Client A and Client B are both "in" (i.e., are occupants of) Room1.
- Client A and Client B both want to be notified when a chat message arrives, so they register a listener function to be executed whenever a message named "CHAT_MESSAGE" is sent to Room1:
room1.addMessageListener("CHAT_MESSAGE", chatMessageListener);
- Client B wants to say hi to everyone in Room1, so it sends an application-defined message named "CHAT_MESSAGE" to Union Server, with a target room of Room1, and the chat text as a message argument:
// Parameters specify: Message Name, Send to Self, Filters, Message Arguments room1.sendMessage("CHAT_MESSAGE", true, null, "Hi");
- Union Server forwards the "chat" message to all clients in Room 1.
- Client A receives the chat message from Union Server, and displays its content on screen.
protected function chatMessageListener (fromClient:IClient, messageText:String):void { incomingMessages.appendText("Guest" + fromClient.getClientID() + " says: " + messageText + "\n"); }
If you understood the preceding code snippets, you'll have no trouble creating amazing realtime connected applications with Union. To get started, follow the steps below.
Making Your Own Union Applications
Here are the typical steps required to create a new Union application.
Step 1: Get Union Server
To build a Union application, you need Union Server. For applications with up to 1000 simultaneous clients, Union Server is free, so you won't need a licence.
There are several ways to get Union Server:
Option 1: tryunion.com
For quick tests and experimentation, many developers use the public Union test server at tryunion.com. No installation or hosting is required, so this option is the quickest way to get a test app up and running.
Option 2: Union Cloud
Sign up for a Union Cloud account. This option is great for developers looking for convenient, reliable Union hosting, or for developers with limited experience running Java applications in a server environment. Union Cloud plans come with Union Server installed, configured, and ready for use.
Option 3: Private Hosting
Download Union Server and run it on your own dedicated or shared server. For private projects, you can also run Union Server on a local LAN. Union Server is quick to set up, but requires some experience with running server-side Java applications. If you plan to run Union on a hosted server, be sure that your hosting provider will allow you to bind to a port. If you expect your application to have over 1000 simultaneous clients, you'll also need to purchase a Union Server Enterprise licence.
Step 2: Pick a Client Framework
Once you have access to Union Server, you can start building your client application with one of the following client frameworks:
Option 1: Orbiter
If you want to make pure web applications that run directly in desktop and mobile web browsers, use Orbiter. Orbiter is a fully functional JavaScript framework, perfect for both tiny multiuser interface widgets and full-fledged browser games and apps. Orbiter's API is identical to Reactor's API, so you can reuse both code and skills across JavaScript and ActionScript projects. To learn how to install and use Orbiter, follow the Orbiter Quick Start.
Option 2: OrbiterMicro
OrbiterMicro is a lightweight configuration of Orbiter, designed for mobile devices and limited-bandwidth environments. To learn how to install and use OrbiterMicro, follow the OrbiterMicro Quick Start.
Option 3: Reactor
If you want to make connected applications for Adobe Flash, use Reactor. Created by Colin Moock, one of the world's leading ActionScript experts, Reactor is a rock-solid, full-featured, mature framework with a rich API and plenty of examples.
Option 4: Build Your Own
Union Platform is designed to allow you, the developer, to create custom clients using your favourite programming language. To learn how to build clients in the language of your choice, see Create a Custom Client.
Step 3: Consider Adding Server-Side Logic
Using Union Platform's powerful client-side API, you can build sophisticated applications without writing a single line of server-side code. But many tasks benefit from server logic. For example, if you're dealing a deck of cards in a game, or maintaining the world state in a physics simulation, you'll want to write some server-side code. To add server-side logic to a Union application, you write room modules and server modules. Server modules add functionality to the entire server. For example, a server module might implement an e-mail-sending function, for use by any client connected to the server. Room modules add functionality to a room. For example, a room module might implement the gameplay logic for a chess game room.
Modules can be written in Java or JavaScript, or any scripting language that has a Java scripting engine (eg. Javascript, Python, Ruby, PHP).
Step 4: Start Coding!
Once your Union Server is running and you've chosen a client framework, you can start coding! These step-by-step tutorials will guide you on your way:
- Orbiter Chat
- Reactor Chat
- Creating a Room Module (for adding server-side logic and functionality)
Step 5: Get Support
You'll find abundant documentation and example code on unionplatform.com. Start with the following resources:
If you have questions or need help, post your issue to the Union forums.
For priority support, consider a Union Member licence.
Have fun!
Creating connected applications is incredibly exciting! If you build something neat, be sure to let us know about it at: union!user1.net (< replace bang with at).
Good luck with your project and happy coding!